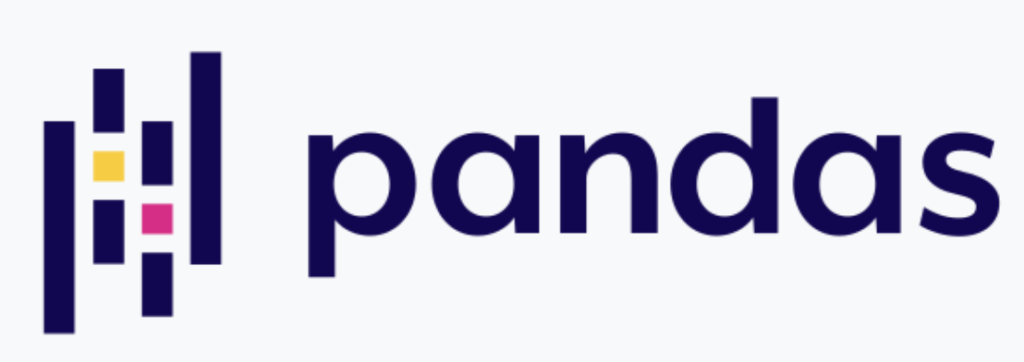
What are the fundamental data structures in pandas and how do they work?
The fundamental data structures in pandas are the Series and DataFrame. A Series is a one-dimensional labeled array capable of holding any data type, while a DataFrame is a 2-dimensional labeled data structure with columns of potentially different types. You can think of it like a spreadsheet or SQL table, or a dict of Series objects. Both data structures have intrinsic data alignment, meaning the link between labels and data will not be broken unless done so explicitly by you. They also accept many different kinds of input, such as dicts, ndarrays, lists, and Series.
How does data alignment work in pandas and why is it important?
Data alignment is intrinsic in pandas, meaning the link between labels and data will not be broken unless done so explicitly by you. This means that when performing operations on pandas objects, such as addition or multiplication, the data will be aligned based on their labels. This is important because it allows for easy manipulation and analysis of data, even when dealing with missing or misaligned values. It also ensures that the results of operations are consistent and accurate.
Can you explain the Series data structure in more detail and provide examples of how it can be used in data analysis?
A Series is a one-dimensional labeled array capable of holding any data type, such as integers, strings, floating point numbers, and Python objects.
The axis labels are collectively referred to as the index. You can create a Series by calling the pd.Series() method and passing in data and index parameters. The data parameter can be many different things, such as a Python dict or an ndarray.
A Series can be used in data analysis for various purposes, such as indexing, slicing, filtering, and mathematical operations. For example, you can use a Series to represent a column of data in a DataFrame or to store time series data. You can also use it to perform calculations on the data or to filter out certain values based on specific conditions.
Here’s an example of how you can create a Series and perform some basic operations on it:
«`
import pandas as pd
# Create a Series with some random data
s = pd.Series([1, 3, 5, 7])
# Print the Series
print(s)
# Output:
# 0 1
# 1 3
# 2 5
# 3 7
# dtype: int64
# Accessing elements by index
print(s[0]) # Output: 1
# Slicing the Series
print(s[1:3]) # Output:
# 1 3
# 2 5
# dtype: int64
# Performing mathematical operations on the Series
print(s * 2) # Output:
#0 2
#1 6
#2 10
#3 14
dtype: int64
«`
In this example, we created a Series with some random integer values and performed various operations on it. We accessed elements by index using square brackets [], sliced the series using colon :, and performed mathematical operations on the series using arithmetic operators.
What is a DataFrame in pandas and how is it different from a Series?
A DataFrame is a 2-dimensional labeled data structure with columns of potentially different types. It can be thought of as a spreadsheet or SQL table, or a dict of Series objects. In other words, it is a collection of Series that share the same index. A DataFrame can be created by passing in a dictionary of arrays, lists, or Series.
On the other hand, a Series is a one-dimensional labeled array capable of holding any data type. It can be thought of as a single column in a spreadsheet or SQL table. A Series has an index that labels each element in the array.
The main difference between a DataFrame and a Series is that a DataFrame has two dimensions (rows and columns) while a Series has only one dimension (rows). A DataFrame can contain multiple columns with different data types, while all elements in a Series must have the same data type.
In summary, while both DataFrames and Series are fundamental data structures in pandas, DataFrames are used to represent tabular data with rows and columns, while Series are used to represent one-dimensional arrays with labels for each element.
How can I read data from a CSV file into a pandas DataFrame?
You can read data from a CSV file into a pandas DataFrame using the `read_csv()` function. Here’s an example:
«`
import pandas as pd
# Read the CSV file into a DataFrame
df = pd.read_csv(‘filename.csv’)
# Print the first 5 rows of the DataFrame
print(df.head())
«`
In this example, we first import the pandas library using `import pandas as pd`. Then, we use the `read_csv()` function to read the CSV file into a DataFrame and assign it to the variable `df`. Finally, we print the first 5 rows of the DataFrame using `print(df.head())`.
The `read_csv()` function has many optional parameters that allow you to customize how you read in your data. For example, you can specify a delimiter other than a comma using the `delimiter` parameter, or skip certain rows or columns using parameters like `skiprows` and `usecols`. You can find more information about these parameters in the pandas documentation.
Can you explain the difference between loc and iloc in pandas?
Yes, I can explain the difference between `loc` and `iloc` in pandas.
`loc` is used to select rows and columns by label. It takes two arguments: the row labels and column labels. You can use it to select specific rows and columns of a DataFrame by their index or label names. For example:
«`
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({‘A’: [1, 2, 3], ‘B’: [4, 5, 6], ‘C’: [7, 8, 9]}, index=[‘a’, ‘b’, ‘c’])
# Select a single row by label
print(df.loc[‘a’])
# Output:
# A 1
# B 4
# C 7
# Name: a, dtype: int64
# Select multiple rows and columns by label
print(df.loc[[‘a’, ‘c’], [‘A’, ‘C’]])
# Output:
# A C
# a 1 7
# c 3 9
«`
On the other hand, `iloc` is used to select rows and columns by integer position. It takes two arguments: the row positions and column positions. You can use it to select specific rows and columns of a DataFrame by their integer position (starting from zero). For example:
«`
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({‘A’: [1, 2, 3], ‘B’: [4, 5, 6], ‘C’: [7, 8, 9]})
# Select a single row by integer position
print(df.iloc[0])
# Output:
# A 1
# B 4
# C 7
# Name:0 , dtype: int64
# Select multiple rows and columns by integer position
print(df.iloc[[0, 2], [0, 2]])
# Output:
# A C
# 0 1 7
# 2 3 9
«`
In summary, `loc` is used to select rows and columns by label, while `iloc` is used to select rows and columns by integer position.
How can I filter rows in a pandas DataFrame based on specific conditions?
You can filter rows in a pandas DataFrame based on specific conditions using boolean indexing. Here’s an example:
«`
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({‘A’: [1, 2, 3], ‘B’: [4, 5, 6], ‘C’: [7, 8, 9]})
# Filter rows where column A is greater than 1
filtered_df = df[df[‘A’] > 1]
# Print the filtered DataFrame
print(filtered_df)
# Output:
# A B C
# 1 2 5 8
# 2 3 6 9
«`
In this example, we first create a DataFrame with three columns (A, B, and C). Then we use boolean indexing to filter the rows where column A is greater than one. We assign the filtered DataFrame to the variable `filtered_df` and print it.
You can use any valid Python expression that returns a boolean value to filter rows in a DataFrame. For example:
«`
# Filter rows where column A is even
filtered_df = df[df[‘A’] %2 ==0]
# Filter rows where column B is between two values
filtered_df = df[(df[‘B’] >3) & (df[‘B’] <6)]
«`
In these examples, we use different conditions to filter the rows of the DataFrame. The first example filters rows where column A is even using modulo operator. The second example filters rows where column B is between two values using logical operators.
In summary, you can filter rows in a pandas DataFrame based on specific conditions using boolean indexing with any valid Python expression that returns a boolean value.
What are some common methods for handling missing data in pandas?
There are several common methods for handling missing data in pandas. Here are some of them:
1. `dropna()`: This method drops any rows or columns that contain missing values. By default, it drops any row that contains at least one missing value.
2. `fillna()`: This method fills in missing values with a specified value or method. For example, you can fill in missing values with the mean or median of the column.
3. `interpolate()`: This method fills in missing values by interpolating between existing values. It can be used to fill in missing values in a time series.
4. `isna()` and `notna()`: These methods return a boolean mask indicating which values are missing (NaN) and which are not.
5. `replace()`: This method replaces specific values (including NaN) with other values.
Here’s an example of how to use these methods:
«`
import pandas as pd
import numpy as np
# Create a DataFrame with some missing data
df = pd.DataFrame({‘A’: [1, 2, np.nan], ‘B’: [4, np.nan, 6], ‘C’: [7, 8, 9]})
# Drop any rows that contain at least one missing value
df_dropped = df.dropna()
# Fill in missing values with the mean of the column
df_filled = df.fillna(df.mean())
# Interpolate between existing values to fill in missing data
df_interpolated = df.interpolate()
# Create a boolean mask indicating which values are NaN
mask = df.isna()
# Replace NaN with a specific value
df_replaced = df.replace(np.nan, -1)
«`
In this example, we first create a DataFrame with some missing data using NumPy’s NaN value. Then we demonstrate how to use each of the five methods listed above to handle the missing data.
Note that the method you choose to handle missing data depends on the nature of your data and the analysis you are performing.
How can I group data in a pandas DataFrame?
You can group data in a pandas DataFrame using the `groupby()` function. The `groupby()` function allows you to group rows of a DataFrame based on one or more columns, and then perform some operation (such as aggregation) on each group. Here’s an example:
«`
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({‘A’: [‘foo’, ‘bar’, ‘foo’, ‘bar’,
‘foo’, ‘bar’, ‘foo’, ‘foo’],
‘B’: [‘one’, ‘one’, ‘two’, ‘three’,
‘two’, ‘two’, ‘one’, ‘three’],
‘C’: [1, 2, 3, 4, 5, 6, 7, 8],
‘D’: [10, 20, 30, 40, 50, 60, 70, 80]})
# Group the DataFrame by column A and calculate the mean of column C for each group
grouped_df = df.groupby(‘A’)[‘C’].mean()
# Print the grouped DataFrame
print(grouped_df)
# Output:
# A
# bar 4.0
# foo 4.6
# Name: C, dtype: float64
«`
In this example, we first create a DataFrame with four columns (`A`, `B`, `C`, and `D`). Then we use the `groupby()` function to group the rows of the DataFrame by column A. We then calculate the mean of column C for each group using the `.mean()` method.
Note that when you use `groupby()`, you get back a new object that is a «GroupBy» object. This object has not actually computed anything yet except for some intermediate data about the groups.
You can also group by multiple columns by passing a list of column names to `groupby()`. For example:
«`
# Group the DataFrame by columns A and B and calculate the mean of column C for each group
grouped_df = df.groupby([‘A’, ‘B’])[‘C’].mean()
# Print the grouped DataFrame
print(grouped_df)
# Output:
# A B
# bar one 2.0
# three 4.0
# two 6.0
# foo one 4.0
# three 8.0
# two 4.0
# Name: C, dtype: float